Laravel 框架
作者:陶冠华
web
什么是框架(Framework)?
框架是实现了通用服务的编程平台。通用服务是指:
1. 验证与授权
2. 安全和加密
3. Cookie和Session
4. 日志
5. 邮件
6. MVC -- Model View and Controller(模型、视图和控制器编程模式)
7. 数据验证
8. 缓存控制
……
使用框架:按规则办事!
学习框架:学习编程规则!
Laravel—— 最受欢迎的PHP框架
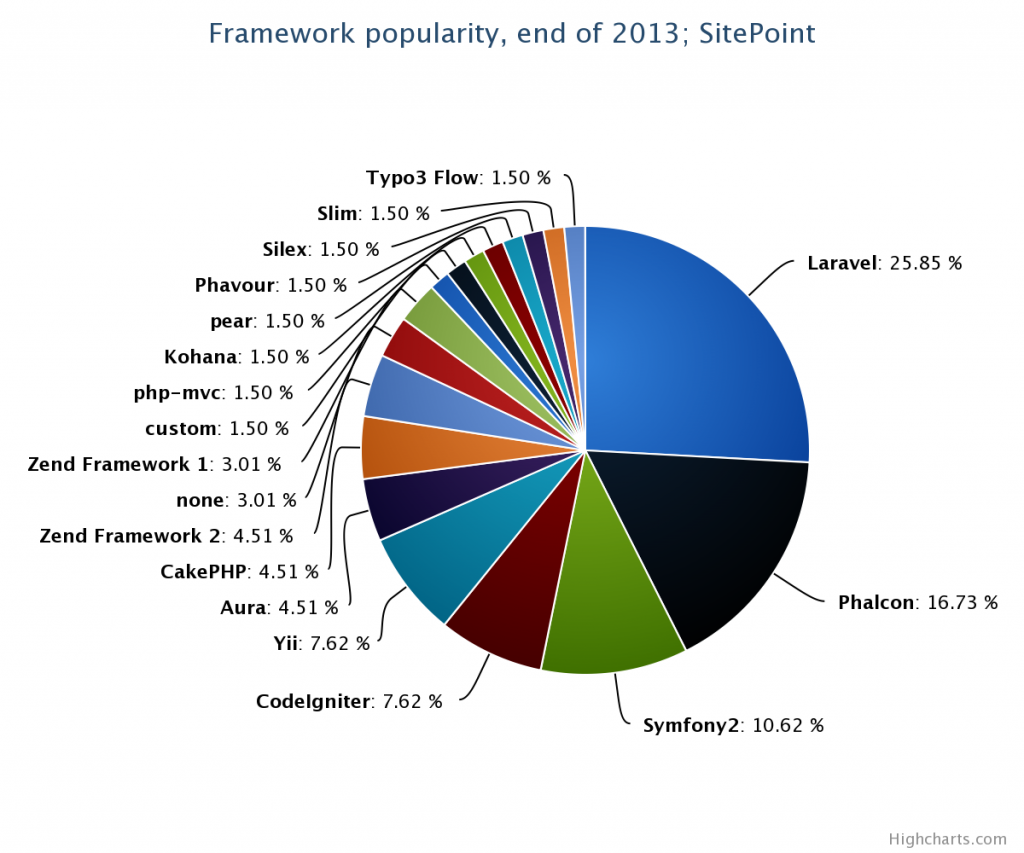
Laravel Philosophy
Laravel is a web application framework with expressive, elegant syntax. We believe development must be an enjoyable, creative experience to be truly fulfilling. Laravel attempts to take the pain out of development by easing common tasks used in the majority of web projects, such as authentication, routing, sessions, and caching.
Laravel aims to make the development process a pleasing one for the developer without sacrificing application functionality. Happy developers make the best code. To this end, we've attempted to combine the very best of what we have seen in other web frameworks, including frameworks implemented in other languages, such as Ruby on Rails, ASP.NET MVC, and Sinatra.
Laravel is accessible, yet powerful, providing powerful tools needed for large, robust applications. A superb inversion of control container, expressive migration system, and tightly integrated unit testing support give you the tools you need to build any application with which you are tasked.
Laravel 的请求-响应过程
浏览器向服务器的public路径发送请求:
http://www.myserver.com/public 或者
http://www.myserver.com/ (将public指定为DocumentRoot)
请求被转到 public/index.php, 在这个文件中,Laravel应用程序对象被创建,各种服务类被载入,请求被转给 app/routes.php;
在app/routes.php中,匹配请求地址的路由被执行,在此可直接将一个视图作为响应返回到浏览器;
- 或者在路由中指出将请求交给某个控制器的某个方法;
- 在这个方法中,可能需要将数据模型从数据库中取出,并在视图中“组装”数据视图,然后返回给浏览器。
Laravel中定义数据模型(实现ORM)
在控制台中进入网站项目根目录,执行:
php artisan generate:migration create_表名_table
- 打开 app/database/migrations/[时间戳]create表名_table.php,在其 up 方法中添加数据字段;
在命令行执行:
php artisan migrate
数据表将会被创建(数据库可在app/config/database.php中配置)
在命令行执行:
php artisan generate:model [模型名]
打开 app/models/[模型名].php,在类中增加以下属性,以构造ORM:
protected $table = "[要映射的数据表名称]";
在Laravel的规范中,如果没有指定以上【要映射的数据表名】,则框架会将“模型名”
变为小写的复数形式去找到“要映射的数据表名” 。如:模型名为:Book时,它会去找到名为books 的数据表作为映射的数据表。
以上实现了ORM。以下步骤用伪数据验证ORM。
6.在命令行执行:
php artisan generate:seed 数据表名
以生成伪数据产生脚本;
7. 打开 app/database/seeds/表名TableSeeder.php, 如:BooksTableSeeder.php;
8. 用Faker对象产生伪数据;
9. 编辑 app/database/seeds/DatabaseSeeder.php, 在其 run 方法中加入:$this->call('表名TableSeeder');
10. 在命令行执行:
php artisan db:seed
以下步骤查看数据。
11. 在一个路由中直接返回数据。如, 在app/routes.php 中:
Route::get('/', function(){
return 数据模型::all();
});
12. 在浏览器地址栏中输入127.0.0.1/mysite/public
(假定项目名称为mysite),可查看到json格式的对象数据。
Eloquent ORM 对象相关的常用方法
- 获取所有对象
模型名::all()
,如:$students = Student::all();
2. 按主键获取对象
模型名::find(主键值或主键值数组)
, 如:theStudent=Student::find(10);students = Student::find(array(1,3,7));
3. 保存对象
对象变量->save()
, 如:$theStudent->save();
4. 删除对象
对象变量->delete()
, 如:$theStudent->delete();
或者:
模型名::destory(主键值或主键值列表)
, 如:Student::destroy(11); Student::destroy(20,21,22);
5. 查找对象
如:Student::where('name','like','张%')->get();
get()中可用一个字段名数组约束返回的属性,如:get(array('id','name'))
6查找并更新对象数据
如:Student::where('city','=','蒙自县')->update(array('city'=>'蒙自市'));
6. 查找并删除数据
如:Student::where('registered','=',0)->delete();
7. 获取某属性的所有取值(不重复)
如:Student::pluck('gender'), 将得到:['男','女'](假定数据中既有男生也有女生)
8. 获取某属性的所有取值(有重复)
如:Student::lists('gender');
Faker 生成伪数据
以下例子生成结果为英语结果,如果要生成不同语言的结果,请在Faker的构造函数中传入本地化名称,如:
$faker = new Faker('zh_CN');
其中:zh_CN 指的是简体中文化名称
一、基本函数
- randomDigit // 生成随机的一位数字,如:7
- randomDigitNotNull // 生成非空的随机的一位数字,如:5
- randomNumber($nbDigits = NULL) // 生成随机数字,参数可指定数字位数,如:79907610
- randomFloat(nbMaxDecimals=NULL,min = 0, $max = NULL) // 生成随机浮点数,如:48.8932,第一位参数指定数字位数,第二个参数指定最小值,第三位参数指定最大值
- numberBetween(min=1000,max = 9000) // 生成某个范围内的随机数字,如:8567。第一位参数指定最小值,第二位参数指定最大值
- randomLetter // 生成随机一位字母,如:'b'
- randomElements(array=array(′a′,′b′,′c′),count = 1) // 从一个数组中随机挑选一个到几个元素构成一个新的数组,如:array('c')。第一个参数指定要挑选元素的数组,第二个参数指定生成新数组中的元素个数
- randomElement($array = array ('a','b','c')) // 从给定数组中随机挑选一个元素,如:'b'。参数指定要挑选的数组
- numerify($string = '###') // 随机生成指定长度的数字字符串,如:'609'。参数指定字符串长度
- lexify($string = '????') // 随机生成一个指定长度的字母构成的字符串,如:'wgts'。参数指定字符串位数
- bothify($string = '## ??') // 随机生成由字母和数字构成的字符串,参数指定格式和位数,如:'42 jz'
二、单词和句子
- word // 生成一个单词,如:'aut'
- words($nb = 3) // 生成单词数组,参数指定单词个数,如:array('porro', 'sed', 'magni')
- sentence($nbWords = 6) // 生成指定单词数的一个句子,参数指定单词数,如:'Sit vitae voluptas sint non voluptates.'
- sentences($nb = 3) // 生成指定句子数量的句子数组,参数指定句子数量,如:array('Optio quos qui illo error.', 'Laborum vero a officia id corporis.', 'Saepe provident esse hic eligendi.')
- paragraph($nbSentences = 3) // 生成一个段落,参数指定句子数量,如:'Ut ab voluptas sed a nam. Sint autem inventore aut officia aut aut blanditiis. Ducimus eos odit amet et est ut eum.'
- paragraphs($nb = 3) // 生成指定段落数量的段落数组,参数指定段落数量,如:array('Quidem ut sunt et quidem est accusamus aut. Fuga est placeat rerum ut. Enim ex eveniet facere sunt.', 'Aut nam et eum architecto fugit repellendus illo. Qui ex esse veritatis.', 'Possimus omnis aut incidunt sunt. Asperiores incidunt iure sequi cum culpa rem. Rerum exercitationem est rem.')
- text($maxNbChars = 200) // 生成指定最大字符数量的文本内容,参数指定最大字符数量,如:'Fuga totam reiciendis qui architecto fugiat nemo. Consequatur recusandae qui cupiditate eos quod.'
三、人
- title($gender = null|'male'|'female') // 生成称谓,如:'Ms.',可在参数中指明男性(male)或女性(female)
- titleMale // 'Mr.'
- titleFemale // 生成女性称谓,如:'Ms.'
- suffix // 生成名字前缀,如:'Jr.'
- name($gender = null|'male'|'female') // 生成姓名,如:'Dr. Zane Stroman',可在参数中指明男性(male)或女性(female)
- firstName($gender = null|'male'|'female') //生成名字,如: 'Maynard'
- firstNameMale //生成男性名字,如: 'Maynard'
- firstNameFemale //生成女性名字,如: 'Rachel'
- lastName //生成姓氏,如: 'Zulauf'
四、地址
- cityPrefix // 生成城市名前缀,如:'Lake'
- secondaryAddress // 生成下级地址,如:'Suite 961'
- state // 生成美国州名,如:'NewMexico'
- stateAbbr // 生成美国州名缩写,如:'OH'
- citySuffix // 生成城市名后缀,如:'borough'
- streetSuffix // 生成街道名后缀,如:'Keys'
- buildingNumber // 生成建筑编号,如:'484'
- city // 生成城市名,如:'West Judge'
- streetName // 生成街道名称,如:'Keegan Trail'
- streetAddress // 生成街道地址,如:'439 Karley Loaf Suite 897'
- postcode // 生成邮政编码,如:'17916'
- address // 生成详细地址,如:'8888 Cummings Vista Apt. 101, Susanbury, NY 95473'
- country // 生成国家名称,如:'Falkland Islands (Malvinas)'
- latitude // 生成纬度,如:'77.147489'
- longitude // 生成经度,如:'86.211205'
五、电话
- phoneNumber // 生成电话号码,如:'132-149-0269x3767'
六、公司
- catchPhrase // 生成广告用语,如:'Monitored regional contingency'
- bs // 生成事业名称,如:'e-enable robust architectures'
- company // 生成对公司名,如:'Bogan-Treutel'
- companySuffix // 生成公司名称后缀,如:'and Sons'
七、文本
- realText(maxNbChars=200,indexSize = 2) // 生成真实文本,如:"And yet I wish you could manage it?) 'And what are they made of?' Alice asked in a shrill, passionate voice. 'Would YOU like cats if you were never even spoke to Time!' 'Perhaps not,' Alice replied."第一个参数指定最大字符数量,第二位参数指定索引大小。
八、日期和时间
- unixTime($max = 'now') // 生成unix格式的时间,如:58781813。参数指定最大时间。
- dateTime($max = 'now') //生成日期时间对象,如: DateTime('2008-04-25 08:37:17'),参数指定最大日期时间。
- dateTimeAD($max = 'now') //生成日期时间对象 DateTime('1800-04-29 20:38:49')
- iso8601($max = 'now') //生成国际标准日期时间字符串,如: '1978-12-09T10:10:29+0000'
- date(format=′Y−m−d′,max = 'now') //生成日期字符串,如: '1979-06-09',第一位参数指定格式,第二位参数指定最大日期时间
- time(format=′H:i:s′,max = 'now') // 生成时间字符串,如:'20:49:42'。第一位参数指定格式,第二位参数指定最大时间。
- dateTimeBetween(startDate=′−30years′,endDate = 'now') // 生成介于某个范围的日期时间对象,如:DateTime('2003-03-15 02:00:49')。第一位参数指定起始日期,第二位参数指定终止日期
- dateTimeThisCentury($max = 'now') //生成本世纪的日期时间对象,如: DateTime('1915-05-30 19:28:21')
- dateTimeThisDecade($max = 'now') //生成本年代的日期时间对象,如: DateTime('2007-05-29 22:30:48')
- dateTimeThisYear($max = 'now') //生成本年度的日期时间对象,如: DateTime('2011-02-27 20:52:14')
- dateTimeThisMonth($max = 'now') //生成本月的日期时间对象,如: DateTime('2011-10-23 13:46:23')
- amPm($max = 'now') // 生成上午下午字符串,如:'pm'
- dayOfMonth($max = 'now') // 生成日子字符串,如:'04'
- dayOfWeek($max = 'now') // 生成星期字符串,如:'Friday'
- month($max = 'now') // 生成月份字符串,如:'06'
- monthName($max = 'now') // 生成月份名称,如:'January'
- year($max = 'now') // 生成年份,如:'1993'
- century // 生成世纪,如:'VI'
- timezone //生成时区,如: 'Europe/Paris'
九、互联网
- email // 生成邮件地址,如:'tkshlerin@collins.com'
- safeEmail // 生成安全的电邮地址,如:'king.alford@example.org'
- freeEmail // 生成免费邮箱,如:'bradley72@gmail.com'
- companyEmail //生成公司电邮,如: 'russel.durward@mcdermott.org'
- freeEmailDomain // 生成免费邮箱域名,如:'yahoo.com'
- safeEmailDomain // 生成安全的邮箱域名,如:'example.org'
- userName // 生成用户名,如:'wade55'
- domainName // 生成域名,如:'wolffdeckow.net'
- domainWord // 生成域名单词,如:'feeney'
- tld // 生成域名后缀,如:'biz'
- url //生成网址,如: 'http://www.skilesdonnelly.biz/aut-accusantium-ut-architecto-sit-et.html'
- slug //生成部分网址,如: 'aut-repellat-commodi-vel-itaque-nihil-id-saepe-nostrum'
- ipv4 // 生成第四版IP地址,如:'109.133.32.252'
- localIpv4 // 生成本地IP地址,如:'10.242.58.8'
- ipv6 //生成第六版IP地址,如: '8e65:933d:22ee:a232:f1c1:2741:1f10:117c'
- macAddress // 生成网络MAC地址,如:'43:85:B7:08:10:CA'
十、浏览器
- userAgent // 生成浏览器名,如:'Mozilla/5.0 (Windows CE) AppleWebKit/5350 (KHTML, like Gecko) Chrome/13.0.888.0 Safari/5350'
- chrome // 'Mozilla/5.0 (Macintosh; PPC Mac OS X 10_6_5) AppleWebKit/5312 (KHTML, like Gecko) Chrome/14.0.894.0 Safari/5312'
- firefox // 'Mozilla/5.0 (X11; Linuxi686; rv:7.0) Gecko/20101231 Firefox/3.6'
- safari // 'Mozilla/5.0 (Macintosh; U; PPC Mac OS X 10_7_1 rv:3.0; en-US) AppleWebKit/534.11.3 (KHTML, like Gecko) Version/4.0 Safari/534.11.3'
- opera // 'Opera/8.25 (Windows NT 5.1; en-US) Presto/2.9.188 Version/10.00'
- internetExplorer // 'Mozilla/5.0 (compatible; MSIE 7.0; Windows 98; Win 9x 4.90; Trident/3.0)'
十一、支付
- creditCardType // 生成信用卡名称,如:'MasterCard'
- creditCardNumber // 生成信用卡号,如:'4485480221084675'
- creditCardExpirationDate // 生成信用卡过期日期,如:04/13
- creditCardExpirationDateString // '生成信用卡过期日期字符串,如:04/13'
- creditCardDetails // 生成信用卡详情数组,如:array('MasterCard', '4485480221084675', 'Aleksander Nowak', '04/13')
十二、颜色
- hexcolor // 生成十六进制颜色,如:'#fa3cc2'
- rgbcolor // 生成红绿蓝三色字符串,如:'0,255,122'
- rgbColorAsArray // 生成红绿蓝三色数组,如:array(0,255,122)
- rgbCssColor // 生成红绿蓝三色CSS,如:'rgb(0,255,122)'
- safeColorName // 生成安全颜色名称,如:'fuchsia'
- colorName // 生成颜色名称,如:'Gainsbor'
十三、文件
- fileExtension // 生成文件扩展名,如:'avi'
- mimeType // 生成媒体种类,如:'video/x-msvideo'
// 从第一个参数指定的位置复制一个随机文件到第二个参数指定的位置,然后返回文件全路径和文件名。
- file(sourceDir=′/tmp′,targetDir = '/tmp') // '/path/to/targetDir/13b73edae8443990be1aa8f1a483bc27.jpg'
- file(sourceDir,targetDir, false) // '13b73edae8443990be1aa8f1a483bc27.jpg'
十四、图像
- imageUrl(width=640,height = 480) // 'http://lorempixel.com/640/480/'
- imageUrl(width,height, 'cats') // 'http://lorempixel.com/800/600/cats/'
- image(dir=′/tmp′,width = 640, $height = 480) // '/tmp/13b73edae8443990be1aa8f1a483bc27.jpg'
- image(dir,width, $height, 'cats') // 'tmp/13b73edae8443990be1aa8f1a483bc27.jpg' it's a cat!
十五、全局唯一标识
- uuid //生成全局唯一标识字符串,如: '7e57d004-2b97-0e7a-b45f-5387367791cd'
十六、条码
- ean13 // 生成13位条码编码,如:'4006381333931'
- ean8 // 生成8位条码编码,如:'73513537'
十七、杂项
1、boolean($chanceOfGettingTrue = 50) // 生成布尔值,参数给定得到“真”值的机会,如:true
2. md5 //生成MD5值,如: 'de99a620c50f2990e87144735cd357e7'
3. sha1 //生成SHA1哈希值,如: 'f08e7f04ca1a413807ebc47551a40a20a0b4de5c'
4. sha256 //生成SHA256哈希值,如: '0061e4c60dac5c1d82db0135a42e00c89ae3a333e7c26485321f24348c7e98a5'
5. locale // 生成本地化名称,如:en_UK
6. countryCode // 生成国家代码,如:UK
7. languageCode // 生成语言代码,如:en